Servo Motor Arduino Code: Best Guide
Working with servo motors through Servo Motor Arduino code is like giving instructions to a robot arm or a little motor that can move precisely to do specific tasks. Imagine it as typing a set of commands on your computer to make something physical happen in the real world.
This is where servo motor Arduino code steps in that helps control these motors, telling them how far to turn, how fast to move, and even where to stop.
Read More About
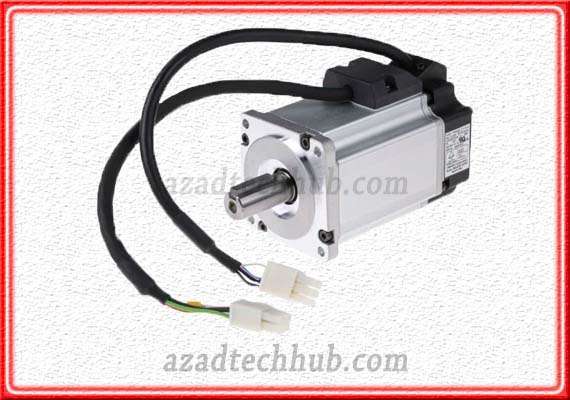
Servo Motor Arduino Code
Servo motor Arduino code acts as a bridge between our ideas and the actual movement of these motors. It’s pretty cool how a few lines of code can bring life to these motors, allowing us to build all sorts of projects, from robotic arms to automated blinds.
With the right code, we can make these motors move smoothly and precisely, opening up a world of possibilities for hobbyists, engineers, and anyone curious about tinkering with technology. So, let’s dive into the realm of servo motor Arduino code and explore the magic it holds in making things move just the way we want them to.
servo motor Arduino code 90 degrees
To control a servo motor with an Arduino to rotate at 90 degrees, you can use the Arduino IDE and the servo library. Here’s a simple example code snippet that you can use:
#include <Servo.h>
Servo myServo; // Create a servo object
void setup() {
myServo.attach(SERVO_PIN); // Replace SERVO_PIN with the pin connected to your servo
}
void loop() {
myServo.write(90); // Rotate the servo to 90 degrees
delay(1000); // Wait for 1 second
}
Replace SERVO_PIN with the pin number to which your servo’s signal wire is connected on the Arduino board. Upload this code to your Arduino, and it will move the servo motor to the 90-degree position and hold it there for 1 second.
Remember, servo motors might have different behaviors, so sometimes, due to mechanical tolerances, the exact angle might vary slightly. Adjustments in the angle can be made by modifying the value in myServo.write() until you achieve the desired position accurately.
servo motor Arduino code 45 degrees
#include <Servo.h>
Servo myServo; // Create a servo object
void setup() {
myServo.attach(9); // Replace 9 with the pin number connected to your servo
}
void loop() {
myServo.write(45); // Rotate the servo to 45 degrees
delay(1000); // Wait for 1 second
}
Replace 9 with the pin number where your servo’s signal wire is connected on the Arduino board. Upload this code to your Arduino, and it will move the servo motor to the 45-degree position and keep it there for 1 second.
Please note that not all servo motors have the ability to move precisely to every degree within their specified range due to mechanical constraints. The accuracy may vary based on the specific servo model and its calibration. Adjustments in the angle can be made by modifying the value in myServo.write() to achieve the closest possible angle for your servo motor.
Arduino ultrasonic sensor servo motor code
Below is an example code that utilizes an ultrasonic sensor and a servo motor with Arduino. This code allows the servo motor to move based on the distance measured by the ultrasonic sensor:
#include <Servo.h>
Servo myServo; // Create a servo object
const int triggerPin = 7; // Trigger pin of the ultrasonic sensor
const int echoPin = 6; // Echo pin of the ultrasonic sensor
void setup() {
Serial.begin(9600);
myServo.attach(9); // Pin 9 is used for the servo motor
pinMode(triggerPin, OUTPUT);
pinMode(echoPin, INPUT);
}
void loop() {
long duration, distance;
digitalWrite(triggerPin, LOW);
delayMicroseconds(2);
digitalWrite(triggerPin, HIGH);
delayMicroseconds(10);
digitalWrite(triggerPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = duration * 0.034 / 2;
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
int angle = map(distance, 0, 200, 0, 180); // Map distance to servo angle range (adjust as needed)
myServo.write(angle); // Move the servo based on distance
delay(500); // Add a small delay before next measurement
}
This code uses an ultrasonic sensor connected to pins 7 (trigger) and 6 (echo) of the Arduino. The sensor measures the distance of an object and calculates it based on the time taken for the ultrasonic pulse to bounce back. Then, it maps this distance to an angle for the servo motor.
Adjust the map() function parameters according to your specific requirements and the range of distances you want to map to the servo angles.
Make sure to properly wire the ultrasonic sensor to the Arduino and the servo motor to the designated pins. Also, note that the actual distance range may vary based on the sensor’s capabilities and the environment.
IR sensor and servo motor Arduino code
Here’s an example code that combines an IR sensor (infrared sensor) with a servo motor using Arduino. This code moves the servo motor based on the detection of an object by the IR sensor:
#include <Servo.h>
Servo myServo; // Create a servo object
int irSensorPin = 2; // IR sensor connected to digital pin 2
void setup() {
myServo.attach(9); // Pin 9 is used for the servo motor
pinMode(irSensorPin, INPUT);
Serial.begin(9600);
}
void loop() {
int irSensorValue = digitalRead(irSensorPin);
if (irSensorValue == HIGH) {
// Object detected by IR sensor
Serial.println("Object Detected!");
myServo.write(90); // Rotate the servo to a specific angle (90 degrees in this case)
delay(1000); // Wait for 1 second
} else {
// No object detected
Serial.println("No Object Detected");
myServo.write(0); // Rotate the servo to another angle (0 degrees in this case)
delay(1000); // Wait for 1 second
}
}
This code assumes the IR sensor is connected to digital pin 2 of the Arduino and the servo motor to pin 9. Adjust the pin numbers according to your actual wiring.
When the IR sensor detects an object (HIGH state), the servo motor moves to a specific angle (here, 90 degrees). When no object is detected (LOW state), the servo motor moves to another angle (0 degrees in this case). You can modify the angles or actions based on your project’s requirements.
Ensure proper connections between the IR sensor and the Arduino, as well as the servo motor’s wiring, for the code to work correctly. Adjust the delays or add more sophisticated logic as needed for your specific application.
servo motor Arduino code 180 degrees
To rotate a servo motor to 180 degrees using Arduino code, you can utilize the servo library. Here’s an example code snippet:
#include <Servo.h>
Servo myServo; // Create a servo object
void setup() {
myServo.attach(SERVO_PIN); // Replace SERVO_PIN with the pin connected to your servo
}
void loop() {
myServo.write(180); // Rotate the servo to 180 degrees
delay(1000); // Wait for 1 second
}
Ensure to substitute SERVO_PIN with the pin number where your servo’s signal wire is connected on the Arduino board. After uploading this code to your Arduino, it will move the servo motor to the 180-degree position and keep it there for 1 second.
However, not all servo motors support a full 180-degree rotation. Some servos might have a limited range, and the physical range can vary based on the specific servo model you’re using. Always refer to the datasheet or specifications of your servo to understand its actual range of movement. Adjust the value in myServo.write() accordingly to suit your servo motor’s actual range.
servo motor Arduino code 360 degrees
Standard servo motors usually have a limited range of motion, typically around 180 degrees. Achieving a full 360-degree rotation with a standard servo motor isn’t possible due to its mechanical limitations.
If you need continuous rotation or a wider range, you might want to consider using a modified servo motor for continuous rotation or a stepper motor that supports full 360-degree rotation.
Continuous rotation servo motors can be controlled to rotate continuously in either direction, effectively providing a full 360-degree range. However, they don’t position at specific angles like standard servos.
For a stepper motor, you’ll require a different approach and specific control using a stepper motor driver and the Arduino stepper library to rotate in steps and achieve a full 360-degree rotation.
If you aim for precise control over a full 360-degree range, consider using a stepper motor or a specialized servo designed explicitly for continuous rotation. Always refer to the datasheet or specifications of your motor to understand its capabilities before attempting to control its movement.
servo motor Arduino code tinkercad
In Tinkercad, you can simulate servo motor control with Arduino using the provided components and code editor. Here’s an example of how you can control a servo motor:
Setup:
- Go to Tinkercad and create a new circuit.
- Search and add an Arduino board and a servo motor from the components panel.
Wiring:
- Connect the servo’s signal pin to a PWM pin on the Arduino (usually marked with ~).
- Connect the servo’s power pin to the 5V pin on the Arduino.
- Connect the servo’s ground pin to the GND pin on the Arduino.
- Ensure your circuit is properly wired.
Code:
- Open the Code Editor for the Arduino component.
- Use the following example code to control the servo motor:
#include <Servo.h>
Servo myServo; // Create a servo object
void setup() {
myServo.attach(9); // Use the pin number where your servo is connected
}
void loop() {
myServo.write(90); // Rotate the servo to 90 degrees
delay(1000); // Wait for 1 second
myServo.write(180); // Rotate the servo to 180 degrees
delay(1000); // Wait for 1 second
}
Simulation:
Click on “Start Simulation” to run the code.
Observe the simulated servo motor moving to the specified angles (90 and 180 degrees) and pausing for one second at each position.
Remember to adjust the pin number in myServo.attach() according to the pin you’ve connected the servo to on the Arduino board in your Tinkercad circuit. This basic code will make the servo motor move between 90 and 180 degrees in the simulation. Adjust the angles and timing as needed for your project.
servo motor Arduino code with potentiometer
To control a servo motor using a potentiometer and Arduino, you can adjust the servo position based on the analog input received from the potentiometer. Here’s an example code that demonstrates this:
#include <Servo.h>
Servo myServo; // Create a servo object
int potPin = A0; // Connect the potentiometer to analog pin A0
void setup() {
myServo.attach(9); // Use the pin number where your servo is connected
}
void loop() {
int potValue = analogRead(potPin); // Read the potentiometer value (0-1023)
int servoAngle = map(potValue, 0, 1023, 0, 180); // Map potentiometer value to servo angle range
myServo.write(servoAngle); // Set the servo position based on the potentiometer value
delay(15); // Add a small delay for stability (adjust as needed)
}
This code uses a potentiometer connected to analog pin A0 of the Arduino board. It reads the analog input from the potentiometer, which varies from 0 to 1023, and maps that range to the angle range of the servo motor (0 to 180 degrees). As you rotate the potentiometer, the servo will move to different positions corresponding to the potentiometer’s analog input.
Make sure to wire the potentiometer correctly, with its outer pins connected to power (5V) and ground (GND), and the middle pin (wiper) connected to analog pin A0.
Adjust the delay time as necessary for your specific setup to ensure smooth and stable servo motor movement based on the potentiometer input.
In wrapping up our dive into servo motor Arduino code, we’ve uncovered a world of possibilities where a few lines of code can make motors spin, turn, and do all sorts of neat tricks.
Working with servo motor Arduino code isn’t just about typing commands; it’s like being a conductor, orchestrating precise movements by telling the motor exactly what to do. It’s fascinating how this code opens doors to creativity, enabling us to build projects where motors move with precision, following our instructions step by step.
Exploring servo motor Arduino code unveils how technology and creativity intertwine. It’s not just about making things move; it’s about understanding how to communicate with machines and bring our ideas to life.
With servo motor Arduino code, we’re not just writing instructions; we’re crafting experiences, making things happen by translating our thoughts into actions that these motors understand. The beauty lies in the simplicity and power of these lines of code, shaping the behavior of servo motors and giving wings to our imagination in the world of Arduino projects.
Frequently Asked Questions
How do I control a servo motor with Arduino?
To control a servo motor with Arduino, you need to use the Servo library. Include the library at the beginning of your code, create a Servo object, and use the attach() function to connect the servo to a specific pin. Then, use the write() function to set the desired angle.
#include <Servo.h>
Servo myServo;
void setup() {
myServo.attach(9); // Connect the servo to pin 9
}
void loop() {
myServo.write(90); // Move the servo to 90 degrees
delay(1000);
myServo.write(0); // Move the servo to 0 degrees
delay(1000);
}
How can I sweep a servo back and forth continuously?
You can use a for loop to sweep the servo back and forth. Adjust the angles and delay times based on your requirements.
#include <Servo.h>
Servo myServo;
void setup() {
myServo.attach(9); // Connect the servo to pin 9
}
void loop() {
for (int angle = 0; angle <= 180; angle += 5) {
myServo.write(angle);
delay(50);
}
delay(1000); // Pause at the end position
for (int angle = 180; angle >= 0; angle -= 5) {
myServo.write(angle);
delay(50);
}
delay(1000); // Pause at the start position
}
How can I control multiple servo motors with Arduino?
You can control multiple servo motors by creating Servo objects for each motor and attaching them to different pins. Then, use the write() function for each servo to set their respective angles.
#include <Servo.h>
Servo servo1;
Servo servo2;
void setup() {
servo1.attach(9); // Connect servo1 to pin 9
servo2.attach(10); // Connect servo2 to pin 10
}
void loop() {
servo1.write(90); // Move servo1 to 90 degrees
delay(1000);
servo2.write(180); // Move servo2 to 180 degrees
delay(1000);
}
Can I use a potentiometer to control a servo?
Yes, you can use a potentiometer to control a servo motor. Connect the potentiometer to an analog pin on Arduino, read the analog input, map the values to servo angles, and use the write() function to control the servo.
#include <Servo.h>
Servo myServo;
int potPin = A0;
void setup() {
myServo.attach(9); // Connect the servo to pin 9
}
void loop() {
int potValue = analogRead(potPin);
int angle = map(potValue, 0, 1023, 0, 180);
myServo.write(angle);
delay(15);
}
Worth Read Posts
- Touch Sensors
- ESP8266 Pinout
- Hysteresis Loss Formula
- ESP32 Pinout
- Work Function Formula
- Power Triangle
- BC547 Pinout
Subscribe to our Newsletter “Electrical Insights Daily” to get the latest updates in Electrical Engineering. You can also Follow us on LinkedIn and Facebook to see our latest posts on Electrical Engineering Topics.