ESP32 Pinout: A Comprehensive Guide to the GPIO Pins
Read More About
Introduction to ESP32
The ESP32 is a powerful and versatile microcontroller board widely used in IoT and embedded systems projects. Understanding the ESP32 pinout, which refers to the arrangement and functionality of the GPIO (General Purpose Input/Output) pins, is essential for effective project development.
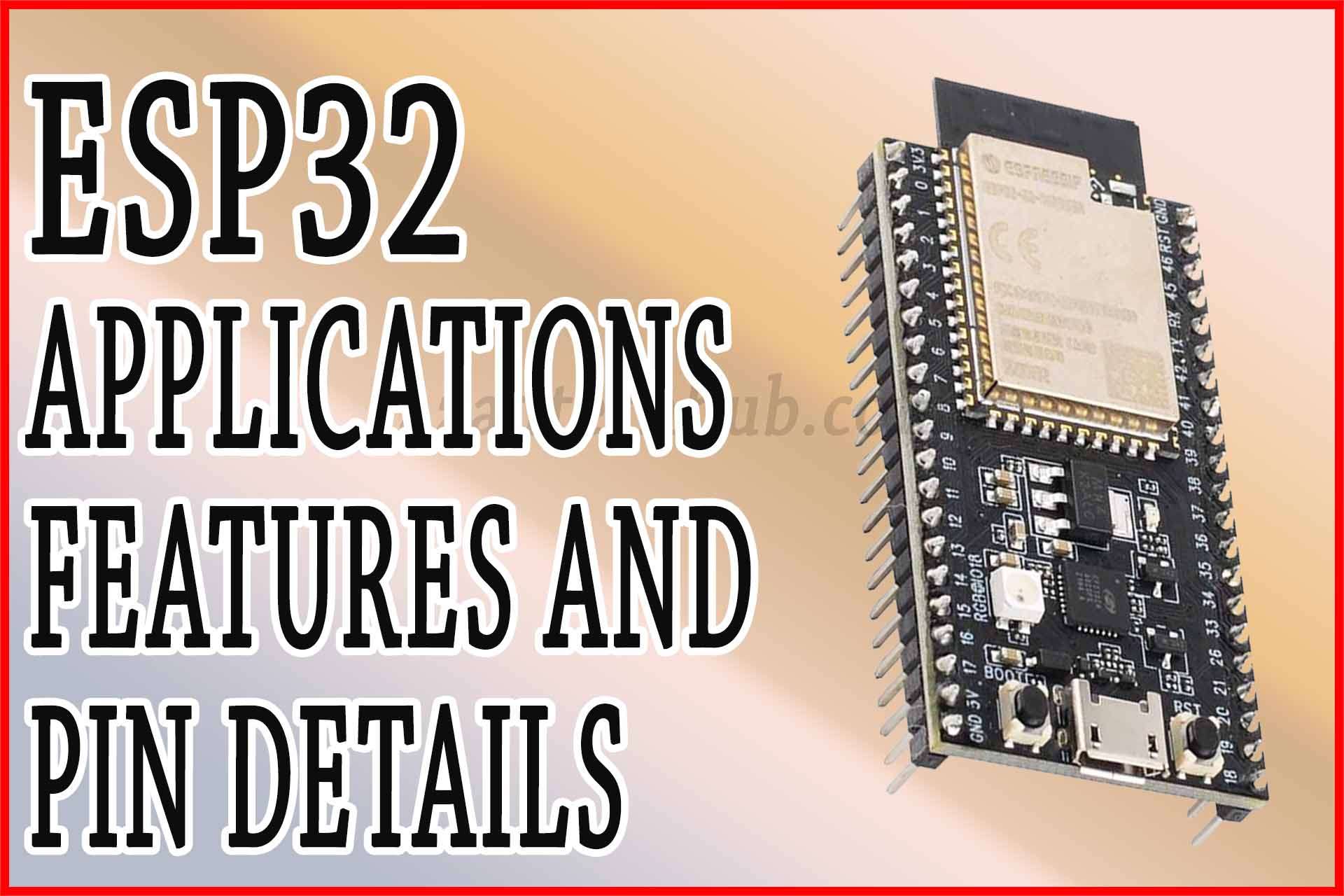
In this article, we will provide a detailed overview of the ESP32 pinout, discussing each pin’s specifications, functionalities, and common use cases. Whether you’re a beginner or an experienced developer, this comprehensive guide will help you unlock the full potential of the ESP32 GPIO pins.
ESP32 Pinout
The ESP32 module comes in various variants, but we will focus on the commonly used ESP-WROOM-32 module, which offers 38 GPIO pins. Below is the detailed description of the ESP32 pinout:
VCC: This pin provides the power supply voltage (typically 3.3V) to the module. Connect a stable power source to this pin.
GND: Connect this pin to the ground of your circuit.
EN: Also known as the Enable pin or the CHIP_EN pin, this pin is used to enable or disable the ESP32 module. To enable the module, connect this pin to the VCC pin.
RST: The Reset pin is used to reset the ESP32 module. Trigger a reset by connecting this pin to the ground momentarily.
GPIO0-GPIO39: The ESP32 module offers 34 general-purpose GPIO pins (GPIO0 to GPIO33) and four input-only GPIO pins (GPIO34 to GPIO39). These pins can be configured as inputs or outputs and used for various purposes in your project.
Analog Input Pins: The ESP32 has multiple analog input pins that can be used to read analog signals from sensors or other analog devices. These pins are labeled as ADC1_CH0 to ADC1_CH7 and ADC2_CH0 to ADC2_CH10.
UART Pins: The ESP32 has multiple UART (Universal Asynchronous Receiver-Transmitter) pins, including TX (transmit) and RX (receive) pins, which enable serial communication with other devices.
I2C Pins: The ESP32 supports I2C (Inter-Integrated Circuit) communication. The I2C pins include SDA (Serial Data Line) and SCL (Serial Clock Line), allowing you to connect and communicate with I2C-compatible devices.
SPI Pins: The ESP32 supports SPI (Serial Peripheral Interface) communication. The SPI pins consist of MOSI (Master Out Slave In), MISO (Master In Slave Out), CLK (clock), and CS (Chip Select). These pins enable communication with SPI devices like sensors, displays, and memory chips.
PWM Pins: The ESP32 provides several pins that support Pulse Width Modulation (PWM) output. PWM pins can be used to control the intensity of LEDs, drive motors, and generate analog-like signals.
Comparison with other Microcontrollers
The ESP32 microcontroller, known for its versatility and connectivity features, has several equivalents or alternatives in the market that offer similar functionalities and capabilities. Here are some microcontrollers that are often considered as alternatives to the ESP32:
ESP8266
- Key Features: Offers Wi-Fi connectivity, GPIO pins, and is cost-effective.
- Use Cases: Ideal for IoT projects and simpler Wi-Fi applications.
- Pros: Cost-effective, widely used in IoT projects.
- Cons: Lacks Bluetooth functionality and has fewer features compared to the ESP32.
Arduino Boards
- Key Features: Wi-Fi/BLE capabilities, compatibility with Arduino ecosystem.
- Use Cases: Great for prototyping and IoT projects.
- Pros: Easy to use with Arduino libraries, good for beginners.
- Cons: Limited processing power compared to the ESP32.
Raspberry Pi Pico
- Key Features: Based on RP2040 chip, GPIO pins, versatile for embedded projects.
- Use Cases: Suited for embedded projects and as a learning platform.
- Pros: Affordable, versatile microcontroller board.
- Cons: Doesn’t have built-in Wi-Fi/Bluetooth.
Particle Boards
- Key Features: Wi-Fi/Cellular connectivity, cloud platform for IoT.
- Use Cases: Ideal for cloud-connected IoT devices and products.
- Pros: Integration with cloud platforms, connectivity options.
- Cons: Cost might be higher for certain applications.
STM32 Series
- Key Features: Offers a wide range of features, uses ARM Cortex-M cores.
- Use Cases: Versatile for embedded systems and various applications.
- Pros: Feature-rich, diverse microcontroller lineup.
- Cons: Learning curve for beginners unfamiliar with ARM architecture.
Microchip AVR/PIC
- Key Features: Various microcontrollers with unique features for automation.
- Use Cases: Embedded projects, automation applications.
- Pros: Diverse microcontroller options.
- Cons: May require specific toolchain for development.
Nordic Semiconductor
- Key Features: nRF52 series known for Bluetooth capabilities.
- Use Cases: Suitable for IoT and wearables due to BLE features.
- Pros: Focus on Bluetooth, suitable for smaller devices.
- Cons: Limited focus on Wi-Fi connectivity.
Example Circuit of ESP32
Let’s provide an example of how you can use the ESP32 pinout in a project:
Example: Temperature and Humidity Monitoring using ESP32 GPIO Pins
In this example, we will demonstrate how to use the ESP32 GPIO pins to interface with a DHT11 temperature and humidity sensor and display the readings on an OLED display. We will be using the ESP-WROOM-32 module.
Components Required:
- ESP-WROOM-32 module
- DHT11 temperature and humidity sensor
- OLED display
- Breadboard
- Jumper wires
Circuit Connections:
Connect the VCC pin of the DHT11 sensor to the 3.3V pin of the ESP32 module.
Connect the GND pin of the DHT11 sensor to the GND pin of the ESP32 module.
Connect the data pin (usually labeled as “OUT” or “DOUT”) of the DHT11 sensor to GPIO pin 4 (D4) of the ESP32 module.
Connect the VCC and GND pins of the OLED display to the 3.3V and GND pins of the ESP32 module, respectively.
Connect the SDA pin of the OLED display to GPIO pin 21 (D21) of the ESP32 module.
Connect the SCL pin of the OLED display to GPIO pin 22 (D22) of the ESP32 module.
Code Example (Using Arduino IDE)
#include <DHT.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define DHTPIN 4
#define DHTTYPE DHT11
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
DHT dht(DHTPIN, DHTTYPE);
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1);
void setup() {
Serial.begin(115200);
dht.begin();
display.begin(SSD1306_SWITCHCAPVCC, 0x3C);
display.display();
delay(2000);
display.clearDisplay();
}
void loop() {
float temperature = dht.readTemperature();
float humidity = dht.readHumidity();
display.clearDisplay();
display.setTextSize(1);
display.setTextColor(SSD1306_WHITE);
display.setCursor(0, 0);
display.print("Temperature: ");
display.print(temperature);
display.println(" C");
display.setCursor(0, 20);
display.print("Humidity: ");
display.print(humidity);
display.println(" %");
display.display();
delay(2000);
}
Explanation of Code
We include the necessary libraries for the DHT11 sensor, OLED display, and I2C communication.
We define the GPIO pins for the DHT11 sensor (D4) and the OLED display (D21 for SDA and D22 for SCL).
In the setup() function, we initialize the DHT11 sensor and OLED display, and clear the display.
In the loop() function, we read the temperature and humidity values from the DHT11 sensor using the readTemperature() and readHumidity() functions, respectively.
We update the OLED display with the temperature and humidity readings using the print() and println() functions.
The display is updated and refreshed every 2 seconds.
By running this code on the ESP32 module, you can monitor the temperature and humidity readings on the OLED display. The GPIO pinout knowledge allows you to correctly connect the sensor and display to the appropriate GPIO pins, ensuring successful communication and accurate data display.
Remember to double check the specific GPIO pin mapping for your ESP32 variant, as it may vary. Additionally, ensure that you have installed the necessary libraries for the DHT11 sensor and the OLED display in your Arduino IDE.
The ESP32 pinout provides a wide range of GPIO pins that can be utilized for various purposes in your projects. This example demonstrates how to interface with a DHT11 sensor and an OLED display using specific GPIO pins.
By understanding the pinout and making accurate connections, you can effectively integrate sensors, displays, actuators, and other peripherals into your ESP32 projects, enabling you to create sophisticated and interactive IoT applications.
Exploring the vast capabilities of the ESP32 GPIO pins opens up endless possibilities for creating innovative projects. Make sure to refer to the pinout diagram and documentation specific to your ESP32 variant to ensure accurate pin mapping and maximize the potential of your ESP32 microcontroller
Conclusion
This comprehensive guide has covered the ESP32 pinout, providing detailed insights into the functionalities and applications of each GPIO pin. Understanding the ESP32 pinout is crucial for successful project development, enabling seamless interfacing with external components.
By leveraging this comprehensive information, you can effectively harness the power of the ESP32 GPIO pins in your IoT and embedded systems projects. Whether you’re a beginner or an experienced developer, this knowledge will empower you to maximize the potential of the ESP32 microcontroller.
Remember to consult the specific pinout diagram or datasheet of your ESP32 variant for accurate pin mapping as it may vary slightly. By accurately identifying and utilizing the GPIO pins, you can connect sensors, actuators, displays, and other peripherals to create a wide range of projects.
Understanding the ESP32 pinout is crucial for successful project development. This detailed guide has provided a comprehensive overview of the ESP32 GPIO pins, explaining their functionalities, specifications, and common applications. By optimizing the utilization of the GPIO pins, you can unlock the full potential of the ESP32 microcontroller in your IoT and embedded systems projects, making them more versatile and powerful.
1. What is the ESP32?
The ESP32 is a versatile microcontroller produced by Espressif Systems, known for its Wi-Fi and Bluetooth capabilities. It’s widely used in IoT, embedded systems, and various electronic projects due to its powerful features and low power consumption.
2. What are the key features of the ESP32?
The ESP32 features a dual-core processor, Wi-Fi and Bluetooth connectivity, a wide range of GPIO pins, analog-to-digital converters (ADC), touch sensors, SPI, I2C, UART, PWM, and more, making it suitable for diverse applications.
3. How does the ESP32 differ from the ESP8266?
The ESP32 is an advanced version of the ESP8266, offering more GPIO pins, Bluetooth connectivity, dual-core processing, lower power consumption, and enhanced capabilities compared to the ESP8266.
4. Can I program the ESP32 using the Arduino IDE?
Yes, the ESP32 can be programmed using the Arduino IDE. Espressif provides a board manager that allows the Arduino IDE to support ESP32, making it convenient for developers familiar with Arduino programming.
5. What programming languages can I use with the ESP32?
The ESP32 can be programmed using various languages, including C/C++, MicroPython, JavaScript (via Espruino), and more. The Espressif IoT Development Framework (ESP-IDF) primarily uses C for development.
6. What kind of projects can I build with the ESP32?
The ESP32’s versatility allows for a wide range of projects, including IoT devices, home automation systems, robotics, sensor networks, smart wearables, data logging applications, and more.
7. Does the ESP32 have built-in Wi-Fi and Bluetooth?
Yes, the ESP32 comes with built-in Wi-Fi and Bluetooth connectivity, enabling easy integration into IoT applications that require wireless communication.
8. How do I power the ESP32?
The ESP32 can be powered via USB using a micro-USB cable or an external power supply. It typically operates at 3.3 volts and can handle a range of input voltages.
9. What is the maximum clock speed of the ESP32?
The ESP32 typically operates at a maximum clock speed of 240 MHz, but it can be adjusted to lower speeds to conserve power.
10. Where can I find resources and support for ESP32 development?
Espressif’s official website, forums like the ESP32 section on the Arduino forum, GitHub repositories, tutorials on platforms like Adafruit, and online communities offer extensive resources, documentation, and support for ESP32 development.
11. Does the ESP32 support OTA (Over-The-Air) updates?
Yes, the ESP32 supports OTA updates, allowing you to upload new firmware to the device remotely, eliminating the need for physical access to update the software.
12. What is the maximum range of Wi-Fi and Bluetooth on the ESP32?
The range for Wi-Fi and Bluetooth on the ESP32 depends on various factors such as the environment, antennas used, and interference. Generally, the Wi-Fi range can extend up to several hundred feet, while Bluetooth can cover shorter distances.
13. Is the ESP32 suitable for battery-powered applications?
Yes, the ESP32 is suitable for battery-powered applications due to its low-power modes and sleep functionalities, which can significantly extend battery life.
14. Can I interface sensors and other devices with the ESP32?
Absolutely, the ESP32’s GPIO pins support a variety of interfaces like I2C, SPI, UART, and analog input, allowing seamless integration with a wide range of sensors and devices.
15. What development platforms or IDEs support ESP32 programming?
Besides the Arduino IDE and ESP-IDF, platforms such as PlatformIO, Espruino, MicroPython, and others provide support for ESP32 development, catering to different programming preferences and needs.
16. Does the ESP32 have built-in security features?
Yes, the ESP32 offers security features like secure boot, flash encryption, and cryptographic hardware accelerators, providing a level of security for IoT and connected devices.
17. Can I create a web server using the ESP32?
Yes, the ESP32 can function as a web server, allowing you to create web-based interfaces to control and monitor devices or display sensor data via a browser.
18. Is the ESP32 compatible with AWS, Azure, or other cloud platforms?
Yes, the ESP32 can be integrated with various cloud platforms like AWS IoT, Azure IoT, Google Cloud IoT, and others, enabling seamless data exchange and control between the device and the cloud.
19. Are there any limitations to consider when using the ESP32?
While the ESP32 is a powerful microcontroller, factors like memory limitations, power consumption, and the number of available GPIO pins for specific functionalities should be considered for complex projects.
20. Where can I find ESP32-based project ideas and tutorials?
Websites like GitHub, Hackster.io, Instructables, and dedicated ESP32 communities on social media platforms often feature a plethora of project ideas, tutorials, and open-source code repositories to inspire and guide your ESP32-based projects.
Worth Read Posts
Subscribe to our Newsletter “Electrical Insights Daily” to get the latest updates in Electrical Engineering. You can also Follow us on LinkedIn and Facebook to see our latest posts on Electrical Engineering Topics.