Arduino Stepper Motor Library: All You Need To Know
The Arduino stepper motor library is a powerful and flexible tool for controlling stepper motors using the Arduino platform. It provides a range of pre-built functions and parameters that make it easy to control the speed, direction, and positioning of stepper motors, and advanced features such as micro stepping, acceleration, and deceleration allow for even greater customization of motor behavior.
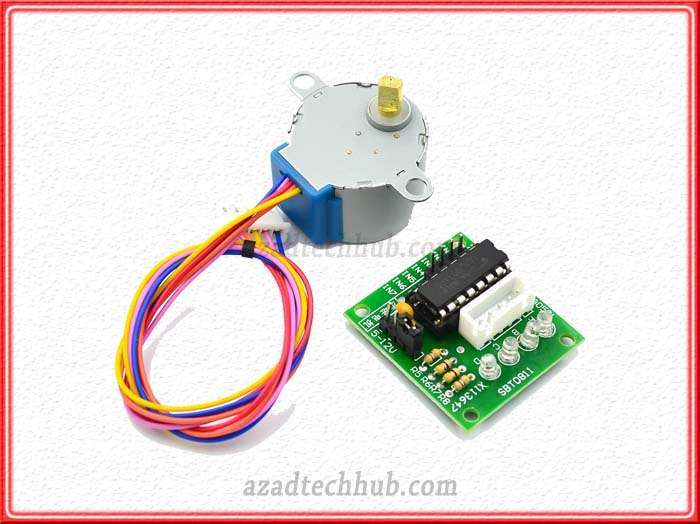
By using the Arduino stepper motor library, you can simplify your programming, achieve smoother and more precise movement, and create motion control systems for a wide range of applications. Whether you’re working on a robotics project, a 3D printer, or a CNC machine, the Arduino stepper motor library provides the flexibility and control you need to get the job done.
Stepper motors are widely used in various applications such as robotics, CNC machines, 3D printers, and more. These motors are known for their ability to precisely control the movement of objects, making them ideal for precise positioning and motion control. The Arduino platform, on the other hand, is a popular open-source electronics platform that enables users to create interactive projects and control devices using software.
To control a stepper motor with an Arduino, you can use the “Arduino stepper motor library”. This library is a powerful tool that simplifies the process of controlling stepper motors, allowing users to focus on the functionality of their projects rather than the intricacies of motor control. In this article, we will explore the Arduino stepper motor library in detail and how it can be used to control stepper motors with ease.
How Arduino stepper motor library Works?
A library is a collection of pre-written code that can be used by programmers to perform common tasks or functions. Libraries are designed to simplify the programming process by providing ready-made code that can be easily incorporated into a program. This reduces the amount of time and effort required to develop complex programs, as programmers can simply use pre-written code to achieve their desired functionality.
An Arduino stepper motor library is a specific type of library that contains functions designed specifically for controlling stepper motors with an Arduino board. This library provides a set of pre-written code that can be used to control the speed, direction, and position of a stepper motor, making it easy for users to implement stepper motor control in their projects.
The Arduino stepper motor library offers several advantages over writing custom code for stepper motor control. First, it saves time by eliminating the need to write code from scratch, allowing users to focus on other aspects of their project. Second, it reduces the risk of errors in the code by providing a set of pre-tested functions that have been proven to work reliably. Finally, it simplifies the learning process by providing an easy-to-use interface that allows even novice programmers to control stepper motors with ease.
Using an Arduino stepper motor library can greatly simplify the process of controlling stepper motors in Arduino projects, providing a reliable and efficient solution for controlling motor movements.
Installing the Arduino Stepper Motor Library
Installing the Arduino stepper motor library is a simple process that can be completed in just a few steps. The following are the steps to install the library using the Arduino IDE:
- Open the Arduino IDE and navigate to the Sketch menu. From there, select Include Library and then Manage Libraries.
- In the Library Manager window, search for “Arduino stepper motor library” in the search bar.
- Select the library from the search results and click on the Install button.
- Wait for the installation process to complete. Once completed, you will see a confirmation message indicating that the library has been successfully installed.
- To use the library in your project, navigate to the Sketch menu and select Include Library. From there, select the Arduino stepper motor library.
- If you encounter any issues during the installation process, the following are some common troubleshooting steps:
- Check that you have the latest version of the Arduino IDE installed.
- Ensure that your internet connection is stable and active during the installation process.
- Verify that the library has been installed correctly by checking for the library in the Installed Libraries section of the Library Manager.
- If you are still experiencing issues, try uninstalling and reinstalling the library or consult the library’s documentation for further assistance.
Installing the Arduino stepper motor library is a straightforward process that can be completed quickly and easily using the Arduino IDE. By following the steps outlined above, you can easily add the library to your project and begin using its functions to control stepper motors with your Arduino board.
How to Use the Arduino Stepper Motor Library
Once you have installed the Arduino stepper motor library, you can begin using its functions to control your stepper motor. The library provides several functions and parameters that you can use to customize the behavior of your motor. Here are some of the library’s most important functions and parameters:
Stepper constructor: This function creates a new instance of a stepper motor, specifying the number of steps per revolution and the pins used to control the motor.
setSpeed() function: This function sets the speed of the motor in revolutions per minute (RPM).
step() function: This function moves the motor a specified number of steps in a given direction.
setAcceleration() function: This function sets the acceleration of the motor, allowing it to ramp up to its target speed gradually.
Here’s an example of how to use the Arduino stepper motor library to control a stepper motor:
Code
#include <Stepper.h>
// Define the stepper motor pins
#define STEPS 200
Then #define DIR_PIN 8
#define STEP_PIN 9
// Initialize the stepper motor object
Stepper motor(STEPS, DIR_PIN, STEP_PIN);
void setup() {
// Set the speed of the motor in RPM
motor.setSpeed(60);
}
void loop() {
// Move the motor 200 steps clockwise
motor.step(200);
delay(500);
// Move the motor 200 steps counterclockwise
motor.step(-200);
delay(500);
}
In this example, we define the pins used to control the motor and create a new instance of the stepper motor object. We then set the speed of the motor and use the step() function to move the motor a specified number of steps in a given direction.
If you encounter issues while using the library, the following are some tips for troubleshooting common issues:
Double-check that you have specified the correct pins for your motor and that they are connected correctly.
Verify that you have set the speed and acceleration of the motor correctly.
Check that your power supply can deliver enough power to your motor.
If your motor is moving erratically or not at all, try adjusting the speed, acceleration, or step count to see if this resolves the issue.
The Arduino stepper motor library provides a simple and efficient way to control stepper motors with an Arduino board. By using the library’s functions and parameters, you can customize the behavior of your motor and create precise and controlled movements in your projects. By following these tips for troubleshooting common issues, you can ensure that your stepper motor is operating as expected.
Advanced Features of the Arduino Stepper Motor Library
In addition to its basic functions, the Arduino stepper motor library also provides several advanced features. That allow you to customize the behavior of your motor even further. These features include micro stepping, acceleration, and deceleration. Here’s a closer look at each of these features:
Microstepping: This feature allows you to subdivide each step of the motor into smaller steps. Providing smoother and more precise movement. The library provides a setMicrostep() function that you can use to set the microstepping mode of your motor.
Acceleration and deceleration: These features allow you to control the speed of the motor smoothly and gradually. Reducing the chances of jerky or abrupt movements. The library provides setAcceleration() and setDeceleration() functions that you can use to set the acceleration and deceleration of your motor.
Here’s an example of how to use these advanced features in code:
Code
#include <AccelStepper.h>
// Define the stepper motor pins
#define STEPS 200
Then #define DIR_PIN 8
#define STEP_PIN 9
// Initialize the stepper motor object
AccelStepper motor(AccelStepper::DRIVER, STEP_PIN, DIR_PIN);
void setup() {
// Set the speed of the motor in RPM
motor.setMaxSpeed(1000);
// Set the acceleration and deceleration of the motor
motor.setAcceleration(200);
motor.setDeceleration(200);
// Set the microstepping mode of the motor to 1/8th
motor.setMicrostep(8);
}
void loop() {
// Move the motor 200 steps clockwise
motor.moveTo(200);
motor.runToPosition();
delay(500);
// Move the motor 200 steps counterclockwise
motor.moveTo(-200);
motor.runToPosition();
delay(500);
}
In this example, we use the AccelStepper library, which is an alternative to the standard Stepper library. It provides additional features such as acceleration and deceleration. We also set the microstepping mode of the motor to 1/8th using the setMicrostep() function. We set the acceleration and deceleration of the motor using the setAcceleration() and setDeceleration() functions. Finally, we use the moveTo() and runToPosition() functions to move the motor a specified number of steps. And wait for it to reach its target position before continuing.
When using these advanced features, there are several tips you can follow to optimize your motor control:
Use the highest microstepping mode that your motor driver and power supply can handle, as this will provide the smoothest and most precise movement.
Experiment with different acceleration and deceleration values to find the optimal balance between speed and smoothness.
When using acceleration and deceleration, be sure to use the moveTo() and runToPosition() functions instead of the step() function, as this will allow the motor to ramp up and down smoothly.
When using high-speed or high-torque motors, be sure to use a power supply that can deliver sufficient current to avoid stalling or overheating.
Benefits of Using an Arduino Stepper Motor Library and Suggestions for Further Learning
The Arduino stepper motor library provides a powerful and flexible way to control stepper motors using the Arduino platform. Some of the benefits of using the library include:
Simplified programming: The library provides pre-built functions and parameters that make it easy to control stepper motors without having to write complex code from scratch.
Customization options: The library provides advanced features such as microstepping, acceleration, and deceleration that allow you to customize the behavior of your motor in a variety of ways.
Increased precision and accuracy: By using the microstepping feature, you can achieve smoother and more precise movement, which can be especially useful in applications such as robotics, 3D printing, and CNC machining.
If you’re interested in exploring the capabilities of the Arduino stepper motor library further, there are several resources available to help you get started. Here are some suggestions:
Check out the official Arduino website, which provides detailed documentation and tutorials on how to use the stepper motor library.
Explore online communities such as the Arduino forum or Reddit’s r/arduino subreddit, where you can ask questions and share tips with other Arduino enthusiasts.
Experiment with different motors and motor drivers to see how they work with the library and to discover new ways to control stepper motors.
Overall, the Arduino stepper motor library is a powerful tool for anyone looking to control stepper motors using the Arduino platform. By taking advantage of its advanced features and customization options, you can create precise and reliable motion control systems for a wide variety of applications.
Subscribe to our Newsletter “Electrical Insights Daily” to get the latest updates in Electrical Engineering. You can also Follow us on LinkedIn and Facebook to see our latest posts on Electrical Engineering Topics.