RN42 Bluetooth Module Arduino: Important Concepts
Using the RN42 Bluetooth module Arduino involves connecting the module to the Arduino board and then programming the Arduino to communicate with the module using the Serial UART interface.
Read More About
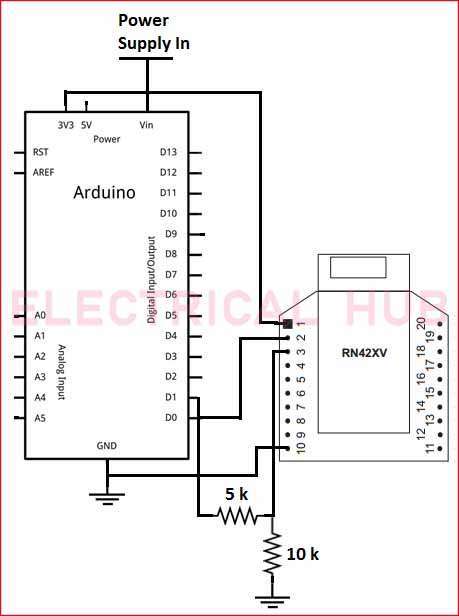
RN42 Bluetooth Module Arduino
Here are the basic steps:
1. Hardware Connections:
Power Supply: Connect the VCC pin of the RN42 module to a 3.3V or 5V power source on the Arduino, and connect the GND pin to the Arduino’s ground.
Serial Communication: Connect the TXD pin of the RN42 module to one of the digital pins on the Arduino (e.g., pin 2) and connect the RXD pin to another digital pin (e.g., pin 3). Ensure that you connect the TXD of the module to the RX pin on the Arduino and vice versa.
Mode Selection: Connect the PIO4 and PIO5 pins of the RN42 module to the ground if you want to operate it in data mode (default mode). If you want to configure the module, connect PIO4 and PIO5 to VCC during power-up.
Optional: LEDs and Buttons: You can add LEDs or buttons to indicate the status or trigger specific actions.
Power Supply Considerations: Ensure that the power supply is within the operating range of the RN42 module, and consider using level shifters if necessary.
2. Software Setup:
Install Arduino IDE: Make sure you have the Arduino IDE installed on your computer.
Install Additional Libraries (if needed): Depending on your project, you might need additional libraries for Bluetooth communication or specific functionalities.
3. Programming the Arduino:
Open the Arduino IDE and create a new sketch.
Use the SoftwareSerial library to create a software serial connection for communication with the RN42 module. Include the library at the beginning of your sketch:
#include <SoftwareSerial.h>
Create a SoftwareSerial object, specifying the RX and TX pins:
SoftwareSerial bluetoothSerial(2, 3); // RX, TX
In the setup function, initialize the serial communication:
void setup() {
Serial.begin(9600); // For communication with the Arduino IDE Serial Monitor
bluetoothSerial.begin(9600); // Set the baud rate to match the RN42 module
}
In the loop function, you can implement the communication protocol with the RN42 module. For example, you can send and receive data:
void loop() {
if (bluetoothSerial.available()) {
char data = bluetoothSerial.read();
Serial.print(data); // Print received data to the Serial Monitor
}
// Your code here
}
Upload the sketch to the Arduino board.
4. Testing:
- Open the Arduino IDE Serial Monitor to view the communication between the Arduino and the RN42 module.
- Ensure that the module is configured correctly for your application.
- Test communication with another Bluetooth-enabled device if required.
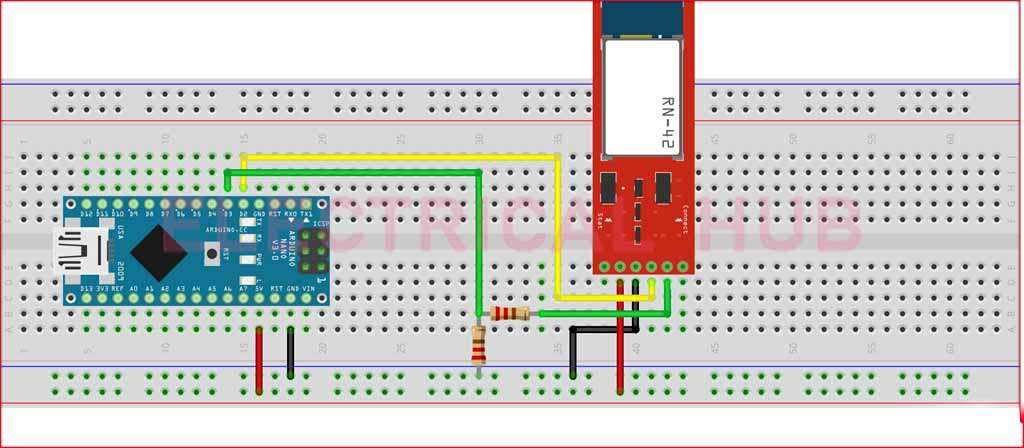
5. Advanced Configuration (Optional):
If you need to configure the RN42 module, refer to the module’s datasheet for the command set and use the Arduino to send the necessary commands.
RN42 Bluetooth Module Arduino Projects
The RN42 Bluetooth module can be integrated into various Arduino projects to add wireless communication capabilities. Here are a few project ideas using the RN42 Bluetooth module Arduino:
Bluetooth Controlled Robot:
Build a small robot controlled via Bluetooth using the RN42 module. Use a mobile app or another Bluetooth-enabled device to send commands to the Arduino, controlling the movement of the robot.
Wireless Sensor Node:
Create a wireless sensor node that collects data from sensors (e.g., temperature, humidity, or motion sensors) and transmits the data to a central Arduino or a Bluetooth-enabled device for monitoring.
Bluetooth Home Automation System:
Design a home automation system where Arduino, equipped with the RN42 module, can control lights, fans, or other devices wirelessly. Use a mobile app to send commands to the Arduino for home automation.
Bluetooth-enabled Weather Station:
Build a weather station using sensors to measure temperature, humidity, and atmospheric pressure. Transmit the collected data to a mobile app or computer via Bluetooth for real-time monitoring.
Bluetooth Game Controller:
Create a wireless game controller using Arduino and the RN42 module. Connect buttons and joysticks to the Arduino, and use Bluetooth to wirelessly control games on a computer or mobile device.
Wireless MIDI Controller:
Build a MIDI controller with knobs, buttons, and sliders connected to an Arduino. Use the RN42 module to wirelessly transmit MIDI data to control music software or instruments.
Bluetooth-enabled Smart Mirror:
Develop a smart mirror that displays information such as weather, calendar events, or news updates. Use the RN42 module to connect the mirror to a mobile app for customization and data updates.
Bluetooth Door Lock System:
Create a Bluetooth-enabled door lock system using Arduino and the RN42 module. Control the locking and unlocking of doors using a mobile app or another Bluetooth device.
Bluetooth-enabled LED Display:
Build an LED display that can be controlled wirelessly using Bluetooth. Display scrolling text, animations, or notifications sent from a mobile app.
Bluetooth-enabled Biometric Access Control:
Develop a biometric access control system that uses fingerprint or facial recognition. Use the RN42 module for wireless communication to grant access or send alerts based on authentication results.
Frequently Asked Questions
What is the RN42 Bluetooth module?
The RN42 is a Bluetooth module that provides Bluetooth connectivity for embedded systems. It supports Bluetooth Classic (2.1) and is designed for easy integration into various applications, including those using the Arduino platform.
How do I connect the RN42 to Arduino?
The RN42 module can be connected to Arduino using serial communication (UART). Connect the TX pin of the RN42 to the RX pin of the Arduino, and the RX pin of the RN42 to the TX pin of the Arduino. Additionally, connect the VCC and GND pins appropriately. Make sure the voltage levels are compatible between the RN42 and Arduino.
How do I configure the RN42 module?
The RN42 module can be configured using simple serial commands. You can communicate with it using a serial terminal on your computer or programmatically through Arduino. The module has default settings, but you may want to configure parameters such as the Bluetooth device name, pairing PIN, baud rate, etc.
Here’s an example of configuring the RN42 with basic commands:
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println(“$$$”); // Enter command mode
delay(1000);
Serial.println(“SM,0”); // Set to slave mode
Serial.println(“SN,MyBluetooth”); // Set Bluetooth name
Serial.println(“SA,0”); // Set security to none
Serial.println(“—“); // Exit command mode
}
void loop() {
// Your main code here
}
How do I send and receive data using the RN42 with Arduino?
Once the RN42 is configured and paired with another Bluetooth device (e.g., computer, smartphone), you can use the Serial library in Arduino to send and receive data. For example, to send data:
void loop() {
Serial.print(“Hello, World!”);
delay(1000);
}
And to receive data:
void loop() {
if (Serial.available() > 0) {
char incomingByte = Serial.read();
Serial.print(“Received: “);
Serial.println(incomingByte);
}
}
Are there any specific considerations for power and voltage?
: The RN42 typically operates at 3.3V. Make sure to provide the appropriate voltage to the module. If you’re interfacing it with a 5V Arduino, use level shifters or voltage dividers to avoid potential damage to the RN42. Check the datasheet for the exact operating voltage range and current requirements.
Can I use the RN42 for wireless communication between Arduino and a smartphone?
Yes, the RN42 can be used for wireless communication between Arduino and a smartphone or any other Bluetooth-enabled device. After configuring the module and establishing a Bluetooth connection, you can exchange data between the Arduino and the connected device.
Worth Read Posts
Subscribe to our Newsletter “Electrical Insights Daily” to get the latest updates in Electrical Engineering. You can also Follow us on LinkedIn and Facebook to see our latest posts on Electrical Engineering Topics.